Hacks Unit 3 Sections 9-11
Home | API | Notes |
Notes
1) Developing Algorithms
When creating an algorithm, its good to outline its process before coding This ensures that it is sequenced correctly You should represent the algorithm using a flowchart or natural language Visualization can help you better see the flow of the whole algorithm This may allow for the coding process to be more efficient and effective
2) Review of Selection and Iteration
Algorithms with iteration repeat a function until a goal is reached To more easily represent an algorithm without showing all the repeated steps, we can use iteration Algorithms with selection only go through certain functions if certain things are true or false
3) Why use algorithms?
When 2 algorithms look extremely similar, it is easy to assume they do the same thing. However, that is not the case and we have learn how to notice small differences in code and pretty much debug.
Hacks 3.9.1
1) Why is it important to know that algorithms that look different can do the same thing and that algorithms that look the same might have different results?
- It is vital to know that algorithms that appear different are capable of performing the same functions, and that algorithms that look the same may have different results. This is because it allows for creativity when coding, leading to finding new or different ways of solving the same issue(s).
2) For the converted conditional to boolean conversion
isSunny = True
isRainy = False
if isSunny == True:
print("No umbrella necessary!")
else:
if isRainy == True:
print("Might want an umbrella!")
else:
print("No umbrella necessary!")
isSunny = False
isRainy = True
# setting variables here (same as above to make comparison easier)
puddles = not(isSunny) and isRainy
if isRainy == False:
print("Puddles!")
if isSunny == True:
print("No puddles!")
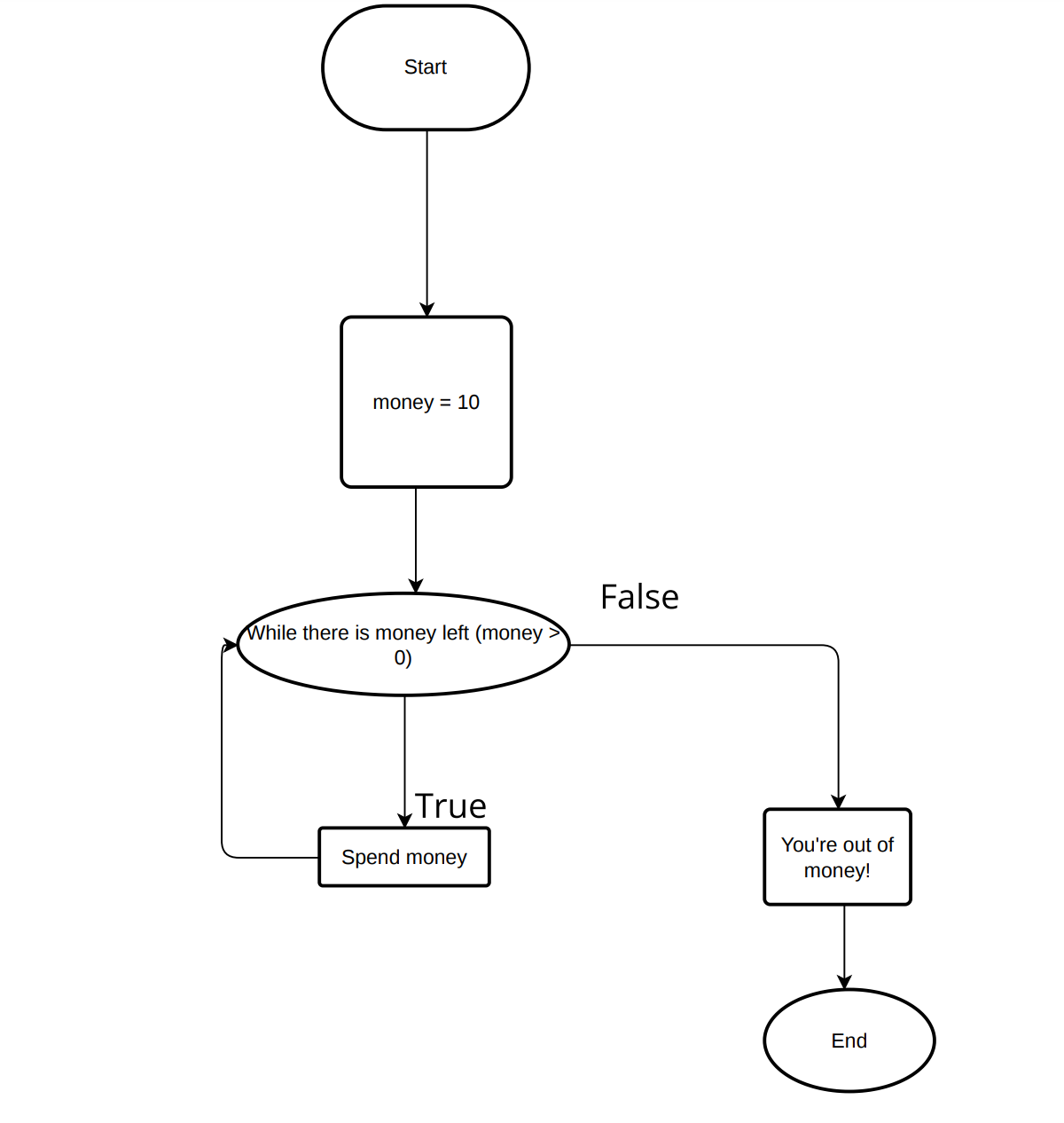
1) Once the code starts, the starting amount of money is set to $10.
2) The variable, spendMoney, is set to True.
3) While spendMoney remains true, $1 is deducted from the money variable.
4) Once the variable, money, reaches a value of 0, spendMoney is set to False and the string, "You're out of money!" is printed.
5) End
money = 10
spendMoney = True
while(spendMoney == True):
money -= 1
if money == 0:
spendMoney == False
print("You're out of money!")
- Fix the number guessing game
1) Make a flow chart for the algorithm number guessing game
2) Make a function that gets the user guess
3) Modify the existing search function to give more encouraging feedback
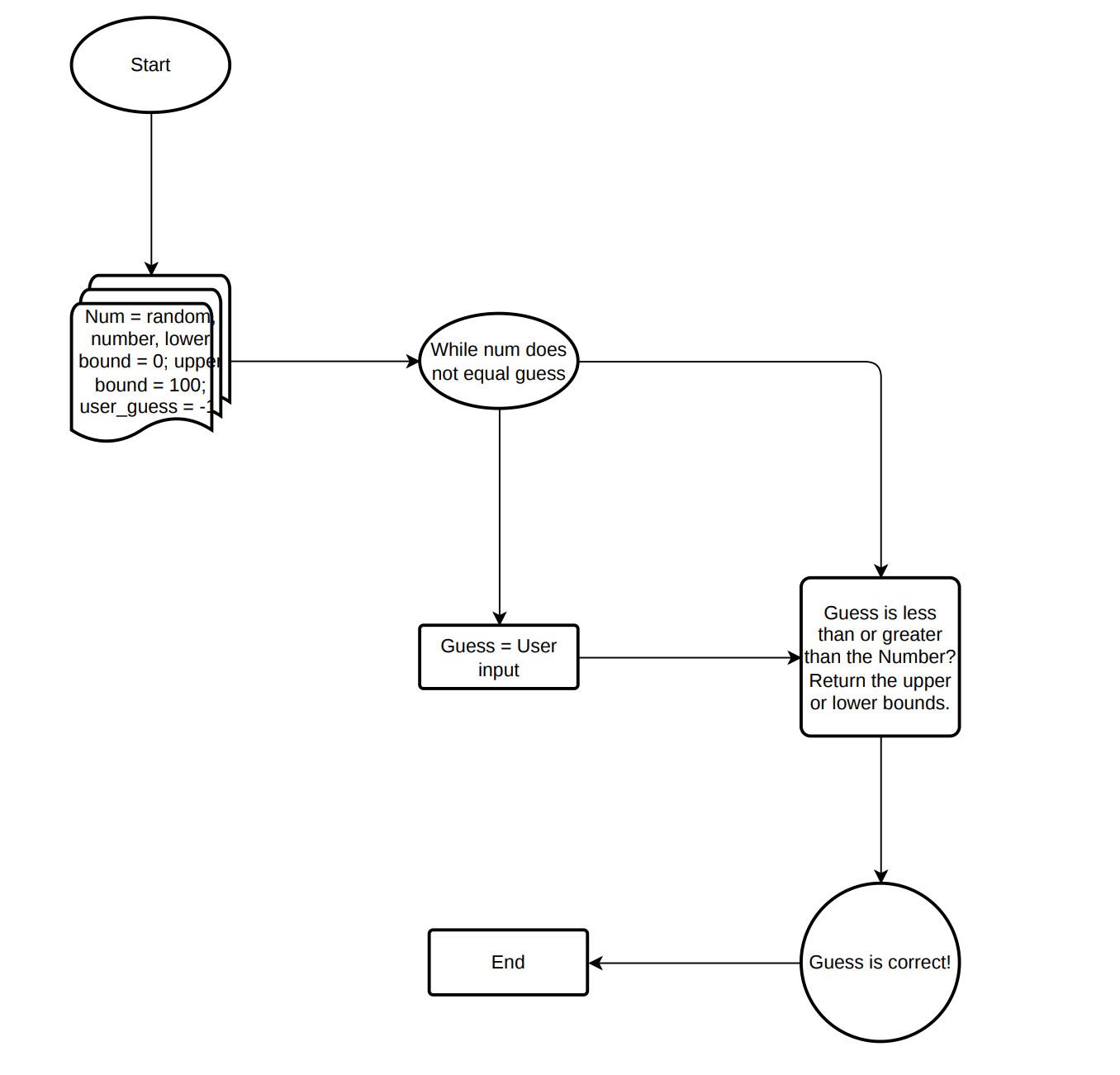
import random
#sets variables for the game
num_guesses = 0
user_guess = -1
upper_bound = 100
lower_bound = 0
#generates a random number
number = random.randint(0,100)
# print(number) #for testing purposes
print(f"I'm thinking of a number between 0 and 100.")
print(number)
#Write a function that gets a guess from the user using input()
def guess():
num = input("Input your guess")
#add something here
return num #add something here
#Change the print statements to give feedback on whether the player guessed too high or too low
def search(number, guess):
global lower_bound, upper_bound
if int(guess) < int(number):
print("Too low, try again! :)") #change this
lower_bound = guess
return lower_bound, upper_bound
elif int(guess) > int(number):
print("Too high, try again! :D") #change this
upper_bound = guess
return lower_bound, upper_bound
else:
upper_bound, lower_bound = guess, guess
return lower_bound, upper_bound
while user_guess != number:
user_guess = guess()
num_guesses += 1
print(f"You guessed {user_guess}.")
lower_bound, upper_bound = search(number, user_guess)
if int(upper_bound) == int(number):
break
else:
print(f"Guess a number between {lower_bound} and {upper_bound}.")
print(f"You guessed the number in {num_guesses} guesses!")
Hacks 3.11
1) calculate the middle index and create a binary tree for each of these lists 12, 14, 43, 57, 79, 80, 99 92, 43, 74, 66, 30, 12, 1 7, 13, 96, 111, 33, 84, 60
Answer: Below
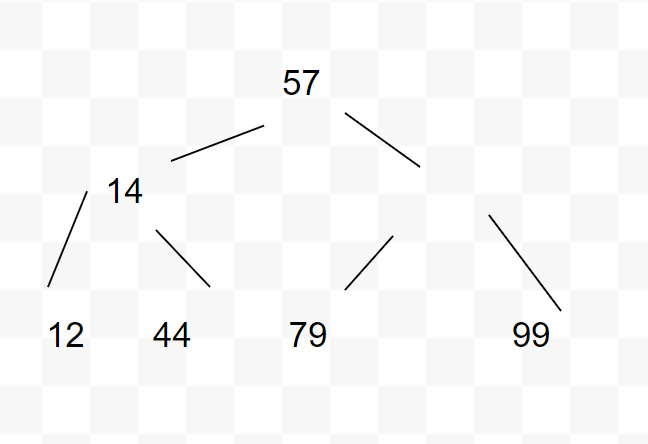
2) Using one of the sets of numbers from the question above, what would be the second number looked at in a binary search if the number is more than the middle number? Set 1: 80, Set 2: 74, Set 3: 96
Answer: Set 1: 80, Set 2: 74, Set 3: 96
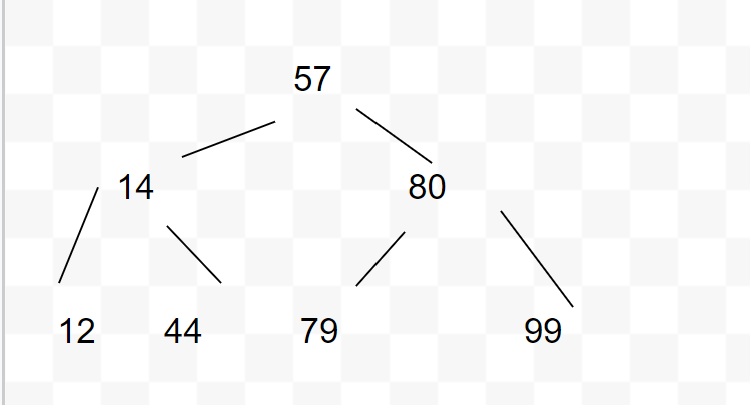
3) Which of the following lists can NOT a binary search be used in order to find a targeted value?
a. ["amy", "beverly", "christian", "devin"]
b. [-1, 2, 6, 9, 19]
c. [3, 2, 8, 12, 99]
d. ["xylophone", "snowman", "snake", "doorbell", "author"]
Answer: C
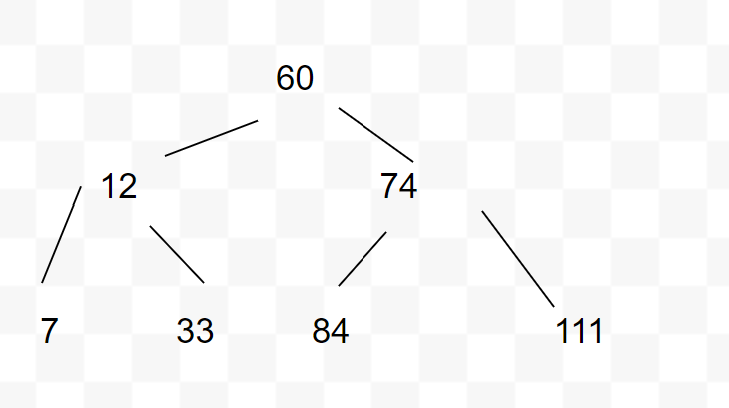
numListOne = [12,14,44,57,79,80,99]
numListTwo = [92,43,74,66,30,12,1]
numListThree = [7,13,96,111,33,84,60]
numLists = [numListOne, numListTwo, numListThree]
for x in range(len(numLists)):
numLists[x].sort()
middle = int(len(numLists[x])/2)
print("Middle Index of List #",x+1,"is",numLists[x][middle])