Hacks Sections 12-13
- Notes
- Section 3.12 Hacks
- Section 3.13 (3.B) Hacks
- Section 3.13 (3.C) Hacks
- Binary Calculator for +, -, *
Home | API | Notes |
Notes
Procedures: A procedure is a block of code that performs a particular task. It can contain variables, constants, data structures, and other programming constructs. Procedures are also known as subroutines, functions, methods, or routines. They are used to break up large programs into smaller, more manageable pieces.
Parameters: Parameters are values that are passed to a procedure or function. They are used to customize the behavior of the procedure or function and can be used to modify its input and output.
Return Values: Return values are values that are returned from a procedure or function. They can be used to indicate the success or failure of a procedure or function, as well as return a value or set of values.
Output Parameters: Output parameters are values that are passed out of a procedure or function. They are used to return values from a procedure or function and can be used to modify its output.
1. Define procedure and parameter in your own words & Define Return Values and Output Parameters in your own words
a) Procedure: A block of code that is created to perform a given task - essentially a function.
b) Parameter: A variable that is utilized in a function that enables data to be imported into said function.
2. Paste a screenshot of completion of the quiz
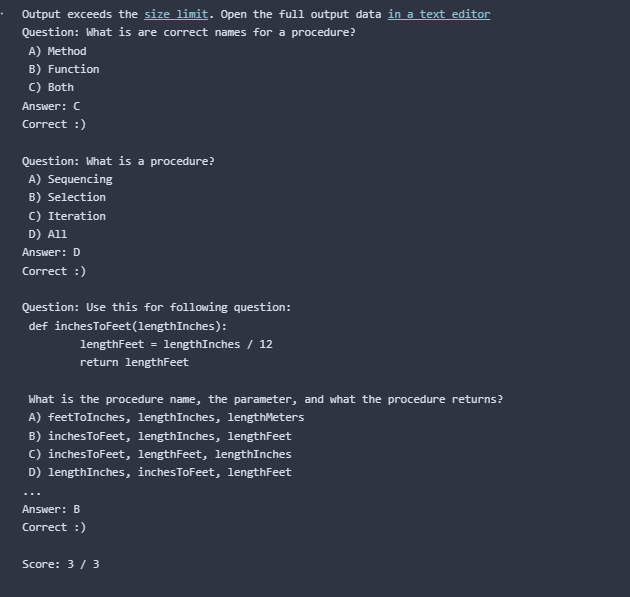
3. Define Return Values and Output Parameters in your own words
a) Return Values: A return value is a value that is returned by a function or method to the calling code after it has finished executing. It is the value that is passed back to the calling code once the function or method has finished its job.
b) Output Parameters: Output parameters are variables that are passed by reference to a function or method and are used to return a value to the calling code. They are typically used to return multiple values from a single function or method.
Code a procedure that finds the square root of any given number. (make sure to call and return the function)
import math
userNum = float(input())
def sqrt(userNum):
return userNum
sqrt = math.sqrt(userNum)
print("Input:" , userNum)
print("Square root:" , int(sqrt))
import math
userNum = float(input())
sqrt = math.sqrt(userNum)
print("Input:" , userNum)
print(sqrt)
Section 3.13 (3.B) Hacks
1) Q: Explain, in your own words, why abstracting away your program logic into separate, modular functions is effective
A: Abstracting away program logic into separate, modular functions is effective when coding because it makes the code easier to read and understand, more reusable and maintainable, and allows for better organization and structure. By breaking down large, complex tasks into smaller, more manageable functions, it can help to simplify the code and make it more organized, which makes debugging and troubleshooting much easier. Additionally, modular functions are easier to update and extend, since they can be tested, modified, and replaced independently. This can help reduce the amount of code needed to be written and improve the overall code quality. Finally, modular functions make code more reusable, as they can be used in multiple projects and functions, which can lead to faster development times and reduced maintenance costs.
2) Create a procedure that uses other sub-procedures (other functions) within it and explain why the abstraction was needed (conciseness, shared behavior, etc.)
The procedure below requires abstraction as it allows the function to organize the code as a whole. Abstracting the variables x and y allows me to not have to call the variables over and over again within the same function.
x = 20
y = 100
# Set function
def addition(x,y):
sum = x + y
return(sum)
#Print the result
print(addition(x,y))
3) Add another layer of abstraction to the word counter program (HINT: create a function that can count the number of words starting with ANY character in a given string -- how can we leverage parameters for this?)
def split_string(s):
# use the split() method to split the string into a list of words
words = s.split(" ")
# initialize a new list to hold all non-empty strings
new_words = []
for word in words:
if word != "":
# add all non-empty substrings of `words` to `new_words`
new_words.append(word)
return words
# this function takes a list of words as input and returns the number of words
# that start with the given letter (case-insensitive)
def count_words_starting_with_letter(words, letter):
count = 0
# loop through the list of words and check if each word starts with the given letter
for word in words:
# use the lower() method to make the comparison case-insensitive
if word.lower().startswith(letter):
count += 1
return count
# this function takes a string as input and returns the number of words that start with 'a'
def count_words_starting_with_a_in_string(s):
# use the split_string() function to split the input string into a list of words
words = split_string(s)
# use the count_words_starting_with_letter() function to count the number of words
# that start with 'a' in the list of words
count = count_words_starting_with_letter(words, "a")
return count
# see above
def count_words_starting_with_d_in_string(s):
words = split_string(s)
count = count_words_starting_with_letter(words, "d")
return count
def any_count(sentence, letter):
words = split_string(sentence)
count = count_words_starting_with_letter(words, letter)
return count
userLetter = input("Type any letter")
answer = count_anything(s, str(userLetter))
print(str(answer) + " word(s) starting with " + str(userLetter))
Section 3.13 (3.C) Hacks
1) Define procedure names and arguments in your own words. Procedures and arguments are used to describe chunks of code that accomplish specific tasks. A procedure name is a label used to identify the code, while arguments are the inputs that the procedure can accept.
2) Code some procedures that use arguments and parameters with Javascript and HTML (make sure they are interactive on your hacks page, allowing the user to input numbers and click a button to produce an output)
-
Add two numbers
-
Subtract two numbers
-
Multiply two numbers
-
Divide two numbers