Hacks Sections 5-7
Home | API | Notes |
Notes
- Boolean: A denoting a system of algebraic notation used to represent logical arguments
- Relational Operators: The mathematical relationship shared between two given variables. Determines whether a statement is true or false based on the output.
- Conditionals: Allow the expression of an algorithms that utilize selection to occur without the use of a programming language.
- Conditional Statement: A statement that affects the sequence of control by executing certain statements depending on the value of a boolean.
Unit 3.5 Hacks
Explain in your own words what each logical operator does NOT: Outputs the opposite of the given data and is typically used for true or false statements - has no effect on the variable. AND: Evaluates two separate conditions and determines if they are all met. OR: Checks for if only one condition is met.
Code your own scenario that makes sense for each logical operator
Tru = True
equals = "="
opposite = not(Tru)
print(opposite)
fun = "fun"
awesome = fun
if fun and awesome == "fun":
print("AND")
else:
print("NOT AND")
import random
numList = ["1", "2", "3", "4", "5"]
ranNum = random.randrange(len(numList))
print(ranNum)
if ranNum == 2 or ranNum == 5:
print("OR")
else:
print("NOT OR")
Unit 3.6 Hacks
- 1 point for defining all the key terms in your own words. 0.5 points if you use examples that show you truly understand it.
- 1 point for writing a program that uses binary conditional logic. 0.5 points if it is original and shows complexity
1) Selection: A construct in which the code will only run if a given condition is met.
- Example: If an answer is true, then the program will run 2) Algorithm: A procedure utilized for completing mathematical operations or problem solving.
- Example: An algorithm that takes in the correct and incorrect (overall points) of a test/quiz, that then displays the users' score. 3) Conditional statement: When a program only runs if a set of conditions are met.
- Example: a) If the basketball is not flat, the game will proceed. b) If the basketball is not broken, then the game will be able to continue.
firstNum = int(input("Input your first number."))
secondNum = int(input("Input your second number."))
conditional = input("AND, OR, XOR?").lower()
if conditional == "and":
print("Selection:" , "AND", firstNum, "&", secondNum, "=", firstNum & secondNum)
elif conditional =="or":
print("Selection:" , "OR ", firstNum, "|", secondNum, "=", firstNum | secondNum)
elif conditional =="xor":
print("Selection:" , "XOR", firstNum, "^", secondNum, "=", firstNum ^ secondNum)
else:
print("Error")
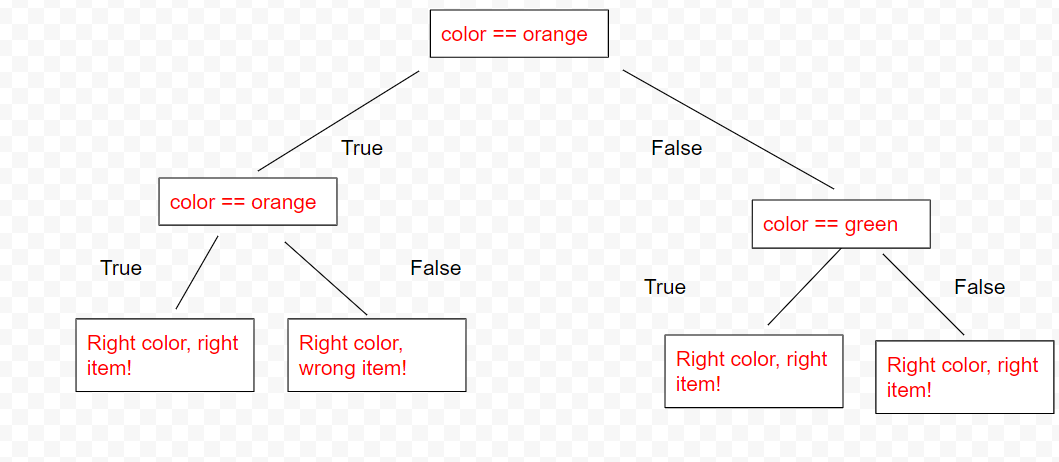
color = "orange"
item = "fruit"
if color == "orange":
print("Right color, wrong item!")
else:
color != "orange" , item == "fruit"
print("Wrong color, right item!")
if color =="green":
print("Wrong color, wrong item!")
else:
item != "fruit" , color != "orange"
print("Right item, right color!")
print(color)
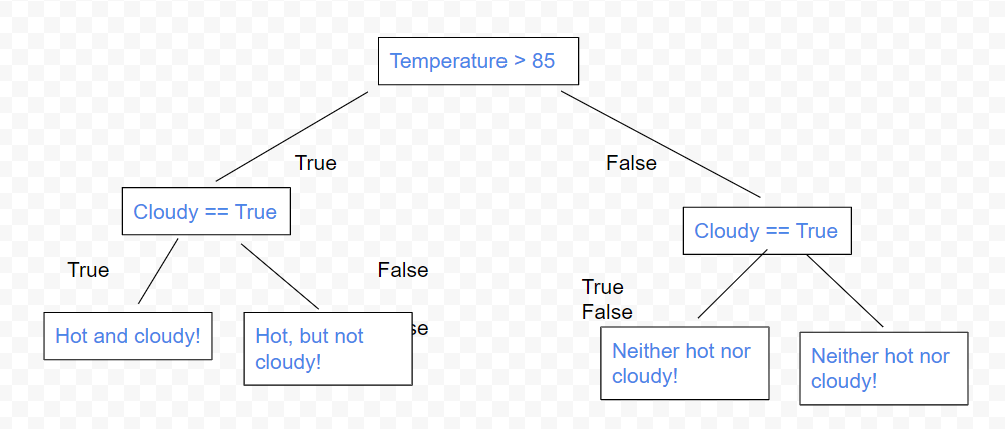
Temperature = 85
Cloudy = True
if Temperature > 85:
if Cloudy == True:
print("Hot and cloudy!")
elif Cloudy == False:
print("Hot, but not cloudy!")
elif Temperature < 80:
if Cloudy == True:
print("Neither hot nor cloudy!")
elif Cloudy == False:
print("Neither hot nor cloudy!")
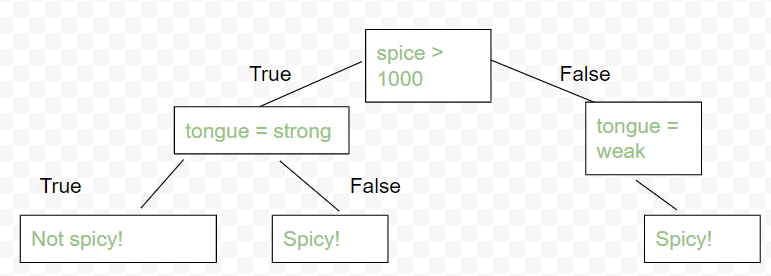
if spice > 1000:
if tongue == "strong!":
print("Not spicy!")
elif milk == "present":
print("Not spicy!")
elif tongue == "weak":
print("Spicy!")
else:
print("Not spicy at all!")
Create a piece of code that displays four statements instead of three. Try to do more if you can.
make = "BMW"
model = "M4 Competition"
year = "2022"
if make == "BMW":
print(make)
if model == "M4 Competition":
print(model)
if year == "2022":
print(year)
print("Nice car!")
Make piece of code that gives three different recommendations for possible classes to take at a school based on two different conditions. These conditions could be if the student likes STEM or not.
STEM = True
MISC = True
if STEM:
print("Algebra, AP CSP, AP Biology")
elif PE:
print("Art, Racket sports, Photography")
else:
print("PE")