Hacks Sections 1-2
Home | API | Notes |
Notes
Essential Knowledge Problems from College Board 1) A variable is an abstraction inside a program that holds a value, where each variable has associated data storage that represents a single value at a time (However, if the value is a collection type such as a list, then the value can contain multiple values). 2) Variables typically have meaningful names that helps with the overall organization of the code and understanding of what is being represented by the variables 3) Some programming languages provide a variety of methods to represent data, which are referenced using variables 9Booleans, numbers, lists, and strings) 4) One form of a value is better suited for representation than another.
Types of Data
- Integers (numbers)
- String (text/letters)
- Boolean (True/False statements)
str="Hello World"
integerOne=7
integerTwo=5
print(str)
print(integerOne + integerTwo)
Unit 3.1.2 Hacks
Prompt: In your own words, briefly explain by writing down what an assignment operator is.
- An assignment operator is a type of operator used to assign a value to a variable. It takes the form of "=", where the value on the right side of the operator is assigned to the variable on the left side. For example, a = 5 assigns the value of 5 to the variable a.
- In Collegeboard pseudocode, what symbol is used to assign values to variables?**
- An arrow (<–) is used to assign values to variables.
A variable, x, is initially given a value of 15. Later on, the value for x is changed to 22. If you print x, would the command display 15 or 22?
- If the variable x was changed to 22, then, print(x) would display 22.
Unit 3.2.1 Hacks
1) What is a list? A list is a sequence of elements in which each element is represented by variable.
2) What is an element? A basic unit of data in a programming language.
3) What is an easy way to reference the elements in a list or string? An easy way to reference the elements in a list or string is to use indexes.
4) What is an example of a string? An example of a string is a sequence of characters such as "Apples".
Unit 3.2.1 Hacks
Create an index of your favorite foods Tips: Index starts at 1, Strings are ordered sequences of characters
Extra work: Try to create an index that lists your favorite food and print the element at index 3. More work: Create a list of your favorite foods and create an index to access them.
marks = [“food1”]
yumyum = ["potatoes", "rice", "pasta", "fried chicken"]
print(yumyum[1])
print(yumyum[3])
num1=input("Input a number. ")
num2=input("Input a number. ")
num3=input("Input a number. ")
add=input("How much would you like to add? ")
# Add code in the space below
numlist = [int(num1), int(num2), int(num3)]
print("User submitted numbers", numlist)
print("Plus " + add)
# The following is the code that adds the inputted addend to the other numbers. It is hidden from the user.
for i in range (len(numlist)):
numlist[i-1] += int(add)
print("Result: ", numlist)
Unit 3.2.3 Hacks
On a single markdown file:
- Insert a screenshot of your score on the python quiz
- Insert a screenshot of your simplification of the food list
Why are using lists better for a program, rather than writing out each line of code? Lists help to organize code and reduce repetitiveness in a program.
Make your own list the “long and slow way” then manage the complexity of the list
Python Quiz Result
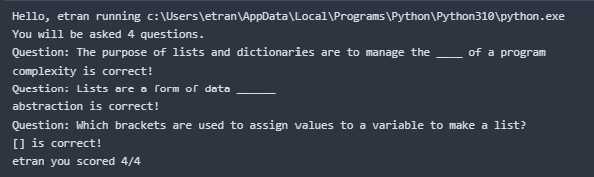
vegetable1 = "Carrots"
vegetable2 = "Broccoli"
vegetable3 = "Cabbage"
vegetable4 = "Radishes"
print(vegetable1, vegetable2, vegetable3, vegetable4)
fruits = ["Apples", "Bananas", "Watermelon", "Oranges"]
print(fruits)