Feature Outline
This is the outline for my car favoriting feature, based on College Board's rubric and criteria
- Row 1 - Program Purpose and Function:
- Row 2 - Data Abstraction:
- Row 3 - Managing Complexity:
- Row 4 - Procedural Abstraction:
- Row 5 - Algorithm Implementation:
- Row 6 - Testing:
- Video Demonstration Considerations
Home | API | Notes |
Overview
- The feature that I will use from our team’s project for the Create Performance task is the overall favoriting system of vehicles on our website.
- My feature will allow users that are signed in to click a heart right beside a desired car or cars
- Once they favorite a car(s) from our site’s inventory or optimal car quiz, they will be able to click the profile tab and view the cars in which they have favorited.
- All of the cars that have been favorited will be stored in a database within the backend, which we plan to set up on AWS (Amazon Web Services) via a Flask server.
- This feature will have use between Mati’s login system, Taiyo’s inventory system, and Luna’s “Optimal Car Quiz”
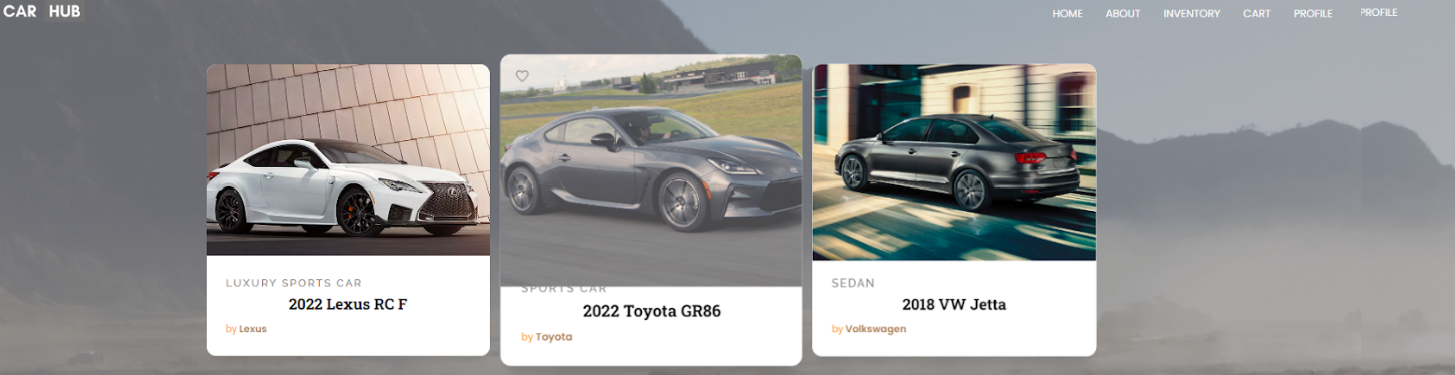
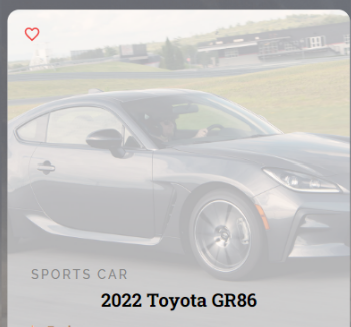
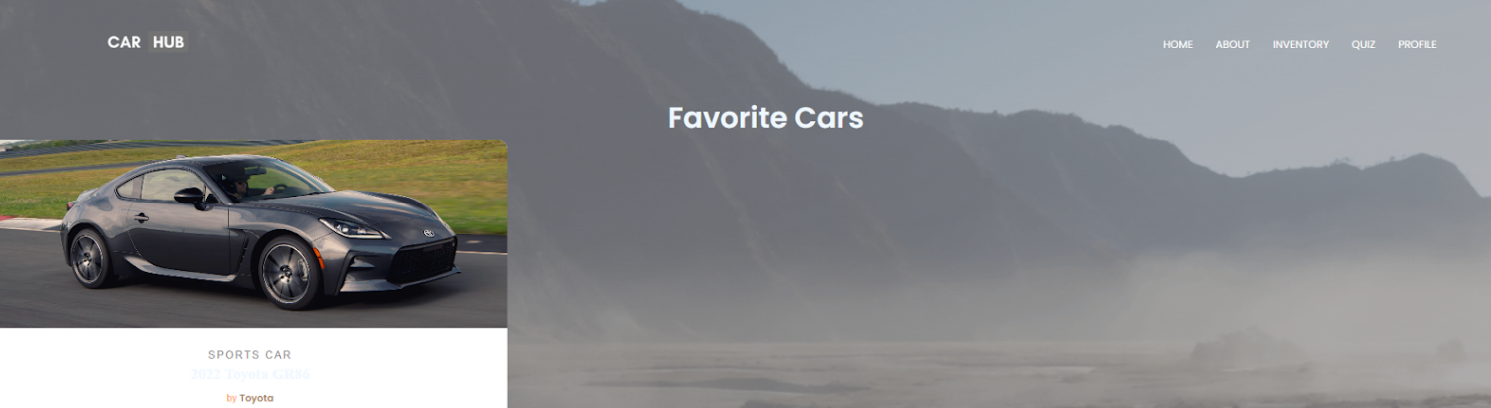
Row 1 - Program Purpose and Function:
- Purpose: The purpose of my program is to allow users to keep track of the cars that they like the most, or fits their needs the best
- Function: The function of my feature is to let users favorite cars and be able to view them via their personal profile - clicking a heart that is displayed on the top left of each car’s profile will enable this feature.
- Input: The sole input required for my feature is the mouse click of the user, which they will use to favorite a given car
- Output: The output of my program is the display of a given user’s favorite car or cars, which they will input through manually clicking the heart on the top left of the car’s profile.
Row 2 - Data Abstraction:
- First code segment: The first program code segment will show a user’s favorited cars within a database (I will implement the database in the future)
- Second code segment: The data in the database will be used for display under our site’s “profile” page/tab. On this page, users will be able to view their unique collection of favorite cars.
- Variable: The name of the variable in the list will be “favoritesCollection”
- Representation: The data in the list represents the given user’s collection of favorite cars
Row 3 - Managing Complexity:
- Lists: Each user’s favorite cars will be stored in a list. These lists will be stored, managed, and retrieved from our database
- Explanation: This data will be under the “favoritesCollection” variable. Without these lists, the program would not function properly, as each user's collection of favorite cars would not be properly stored - thus, the data will not be properly displayed on our website.
Row 4 - Procedural Abstraction:
- Procedure: A procedure named “add_favorite_car” will take action which takes three parameters: username, car, and the “favoritesCollection” list. The procedure appends the car to the favoritesCollection and prints a confirmation message indicating that the car was added to the user's favorite cars list.
- Contribution: The procedure “add_favorite_car” contributes to the overall functionality of the program by allowing the user to add cars to their favorite cars list. This information can then be stored in a database or used for other purposes in the program.
Row 5 - Algorithm Implementation:
This is my plan for the algorithm I plan to code and implement into my team’s website.
- Iteration:The algorithm will obtain a given user’s username and their associated list of favorite cars from the database. A variable “i” will be initialized to keep track of the current car number being displayed. A for loop will then be used to iterate through each car in the favorite cars list.
- Selection: An if/else statement will be used to determine if the list is empty or not. If the list is empty, print a message indicating that the user has no favorite cars.
- Sequencing: Within the for loop, sequencing will be used to print the car number (i) and car name.
Row 6 - Testing:
- Call 1: Condition tested = The favorite cars list associated with "user1" is not empty. Result = The car names and their respective numbers are displayed for "user1".
- Call 2: Condition tested = The favorite cars list associated with "user2" is empty. Result = A message is printed indicating that "user2" has no favorite cars.
- Explanation: The condition being tested by each call is whether the favorite cars list associated with the given username is empty or not. The result of each call is either the display of the user's favorite cars and their respective numbers or a message indicating that the user has no favorite cars.
Video Demonstration Considerations
My video will have to show my codes input, output, and overall program functionality. This means that my code must function as intended without any mistakes or fatal errors. In my video, I will have to be logged in to an account on our website, which is a part of Mati's feature. I will need to show my feature by favoriting cars from our site's inventory and from my "Optimal Car Quiz" results - Luna's feature. To show that my program properly functions, I will need to go to my personal profile and show the display of my favorite cars based upon my input.